1. Overview of MySQL LIKE
The MySQL LIKE
operator is used to search for data within a database that matches a specific pattern. LIKE
is used in the SQL WHERE
clause to set search conditions based on a part or the whole of a string. For example, it is useful when searching for “names starting with a certain letter” or “product codes containing a specific sequence of characters.”
Uses of the LIKE Operator
- Partial match search
- Specific pattern search
- Data filtering
This operator specializes in pattern matching, making it indispensable for efficiently searching and manipulating data within a database.
2. Basic Syntax of MySQL LIKE
The basic syntax for using the LIKE
operator in MySQL is as follows:
SELECT column_name FROM table_name WHERE column_name LIKE 'pattern';
Examples of Using LIKE
- Searching for data starting with specific characters
SELECT * FROM users WHERE name LIKE 'A%';
- Searching for data containing a specific string
SELECT * FROM products WHERE product_code LIKE '%123%';
The LIKE
operator is used in combination with wildcards such as %
and _
. This allows for more flexible searches.
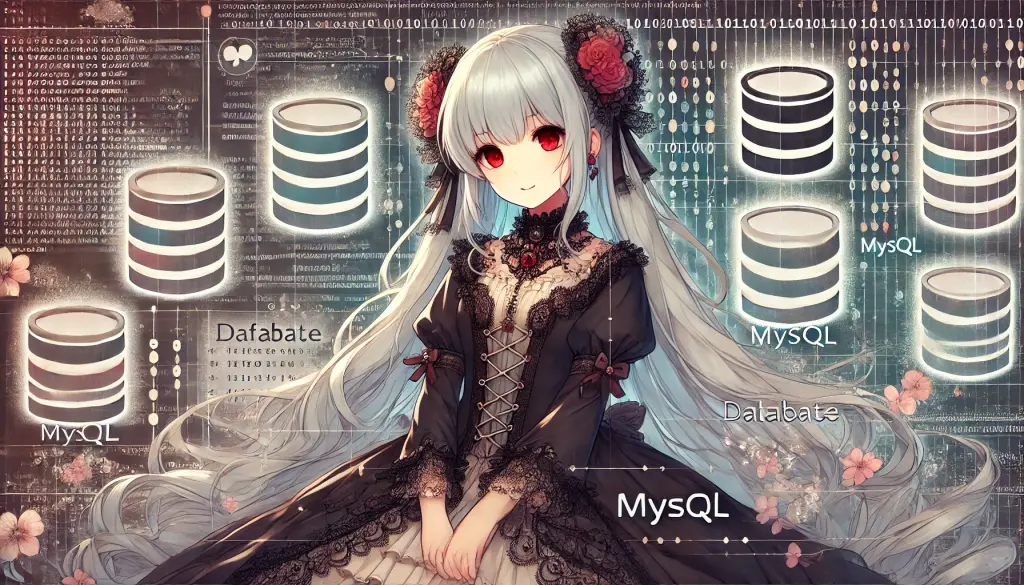
3. Wildcards Used with LIKE
The LIKE
operator uses wildcards to specify search patterns. The main wildcards supported by MySQL are the following two:
The %
Wildcard
- Matches any sequence of characters (zero or more)
SELECT * FROM users WHERE email LIKE '%@example.com';
This example searches for all email addresses ending with@example.com
.
The _
Wildcard
- Matches any single character
SELECT * FROM products WHERE product_code LIKE '_A%';
This example searches for all product codes where the second character isA
.
By using wildcards appropriately, you can efficiently filter data within the database.
4. Pattern Matching Techniques
Various pattern matching techniques are possible by using the LIKE
operator and wildcards.
Starts With
- Searching for data where a string begins with a specific pattern
SELECT * FROM customers WHERE name LIKE 'John%';
This searches for all customer names starting withJohn
.
Ends With
- Searching for data where a string ends with a specific pattern
SELECT * FROM files WHERE filename LIKE '%.pdf';
This searches for all filenames ending with.pdf
.
Contains
- Searching for data where a string contains a specific pattern
SELECT * FROM documents WHERE content LIKE '%MySQL%';
This searches for all documents containing the stringMySQL
.
5. Escaping Special Characters in LIKE
In the LIKE
operator, %
and _
have special meanings as wildcards. To search for these as regular characters, you need to use an escape character.
How to Escape
- Example of searching using an escape character
SELECT * FROM filenames WHERE filename LIKE 'file\_%' ESCAPE '\';
In this query, all filenames starting withfile_
are searched. The_
is usually treated as a wildcard, but by using the escape character\
, it is treated as a regular character.
6. Advanced Usage of LIKE
The LIKE
operator can be combined with other SQL statements for more advanced searches.
Combining with JOIN
- Searching for related data between tables
SELECT orders.id, customers.name FROM orders JOIN customers ON orders.customer_id = customers.id WHERE customers.name LIKE '%Smith%';
This query retrieves orders from customers whose names containSmith
.
Negation with NOT LIKE
- Searching for data that does not match a specific pattern
SELECT * FROM emails WHERE address NOT LIKE '%@spam.com';
This retrieves email addresses that do not end with@spam.com
.
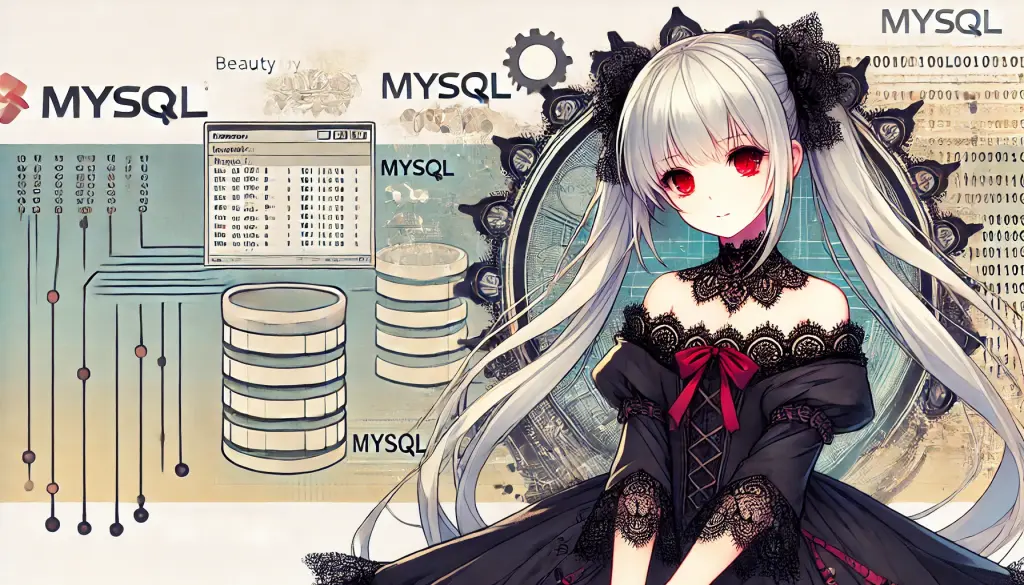
7. Best Practices When Using LIKE
There are several points to note and best practices when using the LIKE
operator.
Impact on Performance
- When using the
LIKE
operator, performance may decrease when searching large amounts of data. Especially when using%
at the beginning of the pattern, indexes may not be effective, causing the query to slow down.
Proper Use of Indexes
- To improve performance, consider creating indexes as needed.
8. Common Uses of MySQL LIKE
The MySQL LIKE
operator is used in various scenarios, such as:
Customer Search
- When performing searches based on customer names or email addresses.
Product Code Search
- When searching for products based on a part of their product code.
9. Conclusion
The LIKE
operator is a powerful pattern matching tool in MySQL. This article has broadly explained everything from basic syntax to advanced usage and performance optimization. To efficiently search and manipulate your database, make sure to utilize the LIKE
operator appropriately.
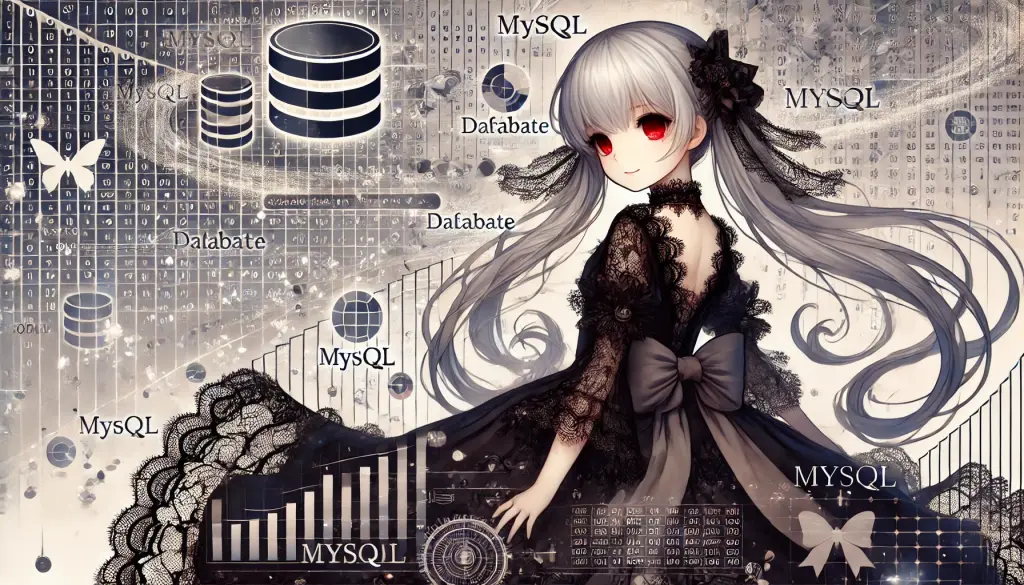
10. Frequently Asked Questions
Q1: What is the difference between LIKE
and =
?
A1: =
is used for exact match searches, while LIKE
is used for partial match and pattern matching.
Q2: Is LIKE
case-sensitive?
A2: In the default MySQL setting, LIKE
is not case-sensitive. However, you can make it case-sensitive by using the BINARY
keyword.
Q3: Can the LIKE
operator be used with numbers?
A3: It is primarily used with strings, but it can be used if numbers are stored as strings.